Code Type
This document is referring to a past Scout release. Please click here for the recent version. |
A CodeType is a structure to represent a tree key-code association. They are used in SmartField and SmartColumn.
-
implements:
ICodeType<T>
-
extends:
AbstractCodeType<T>
Description
CodeTypes are e.g. used in SmartFields, ListBoxes or TreeBoxes to let the user choose between a finite list of values. The value(s) stored by the field correspond to the key(s) of the selected code.
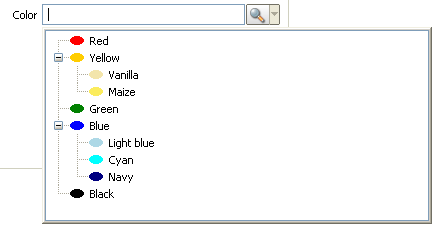
A CodeType can be seen as a tree of Codes. Each code associates to the key (the Id) other properties: among others a Text and an IconId.
In order to have the same resolving mechanism (getting the display text of a key), CodeTypes are also used in SmartColumns. To choose multiple values in the list, the fields ListBox (flat CodeType) and TreeBox (hierarchical CodeType) can be used.
Organisation of the codes
The codes are organized in a tree. Therefore, a CodeType can have one or more child codes at the root level, and each code can have other child codes. Often a flat list of codes (without nested codes) is sufficient to cover most of the needs.
Child codes are ordered in their parent code. This is implemented using the @Order
annotation on the code class.
Type of the key
The type of the key is defined by its generic parameter <T>
. It is very common to use a type from the java.lang.*
package (like Long
or String
), but any Java Object is suitable. It must:
-
implement
Serializable
-
have correctly implemented
equals()
andhashCode()
functions -
be present in the server and the client
There is no obligation to have the same type for the Id between the codes of a CodeType (meaning the same generic type parameter for the codes inner-class). However, it is a good practice to have the same type between the codes of a CodeType, because the Id might be used as value of e.g. a SmartField. Therefore, the generic parameter describing the type of value of a SmartField must be compatible with the type of the codes contained in the CodeType.
Using a CodeType
SmartField or SmartColumn
CodeType in a SmartField (or SmartColumn).
@ClassId("08ccc68e-7b72-4fe0-b666-245ddb8b8441")
public class YesOrNoSmartField extends AbstractSmartField<Boolean> {
// other configuration of properties.
@Override
protected Class<? extends ICodeType<?, Boolean>> getConfiguredCodeType() {
return YesOrNoCodeType.class;
}
}
If the SmartField (or SmartColumn) works with a CodeType, a specific LookupCall is instantiated to get the LookupRows based on the Codes contained in a CodeType.
Accessing a code directly
Scout-runtime will handle the instantiation and the caching of CodeTypes.
This function returns the text corresponding to the key using a CodeType:
public String getCodeText(boolean key) {
ICode c = BEANS.get(YesOrNoCodeType.class).getCode(key);
if (c != null) {
return c.getText();
}
return null;
}
Static CodeType
Java Code and structure
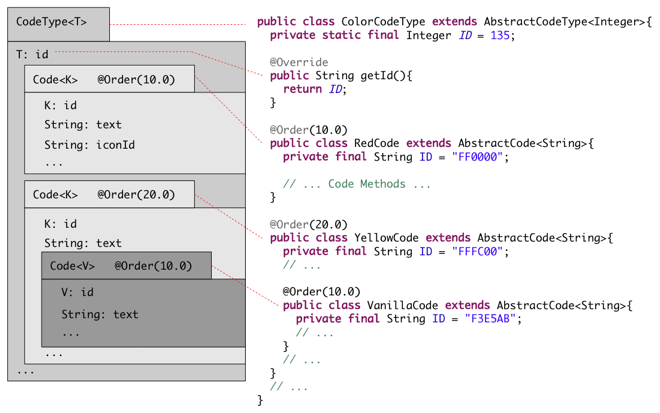
The common way to define a CodeType is to extend AbstractCodeType. Each code is an inner-class extending AbstractCode. Like usual the properties of Codes and CodeTypes can be set by overriding any of the inherited methods starting with getConfigured
.
See the Java Code of a simple YesOrNoCodeType
having just two codes:
-
YesOrNoCodeType.YesCode
-
YesOrNoCodeType.NoCode
Dynamic CodeType
Code types are not necessarily hardcoded. It is possible to implement other mechanisms to load a CodeType dynamically.
The description of the Codes can e.g. come from a database or any other data source. If you want to do so, you just need to implement the method corresponding to the event LoadCodes
.
It is possible to use the static and the dynamic approach together. In this case, if there is a conflict (2 codes for the same id) the event OverwriteCode is triggered.
Note for advanced users:
Each CodeType is instantiated for
-
each language
-
each partition
Note: A CodeType class is instantiated for each Locale and managed by the CodeService
.